Using CSS Grid auto-fit/auto-fill to create dynamic columns
I’m sure that many are familiar with using the Flexbox Albatross to achieve wrapping columns on smaller screens without needing write a media query. Jonathan Snook has actually modified this and created a grid version version of this.
What I’m wanting to setup is an auto filling grid that doesn’t require media queries and can support having some of it’s elements be full width.
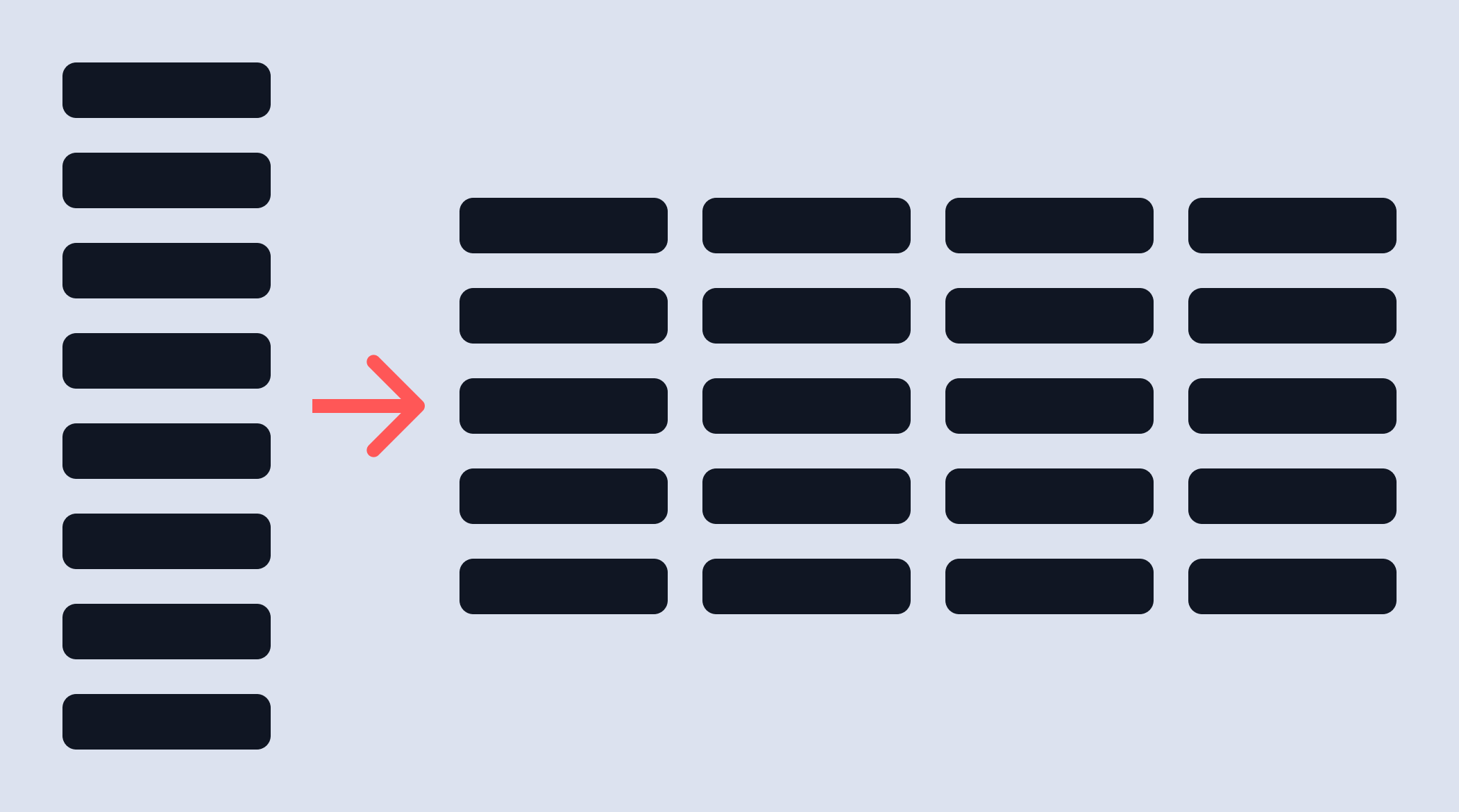
HTML Setup
For the HTML you will need a parent for the grid and then your child columns inside of it. You can then add classes for the modifiers you would like, for this example I am using a full width option and an 2x option.
<div class="grid">
<div class="col col--full">...</div>
<div class="col">...</div>
<div class="col">...</div>
<div class="col">...</div>
<div class="col">...</div>
</div>
CSS Setup
Base grid setup
First we will setup the base grid for the parent wrapper. For this we will use the repeat() function and set the repeat count to auto-fit, I am using auto-fit because I want the columns to grow to fill the available space, if you don’t want them to grow to fill the space then use auto-fill.
Then we will set the tracks, or sizing, using the minmax function. The first size will be 15rem, which will be the minimum size we want the column to be. The second value will be 1fr, which will make all the columns be equal widths.
.grid {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(15rem, 1fr));
}
Column sizing
Now we style the full width modifier class to start at column 1 and end at -1. In this case negative one means that it ends at the last column, which is helpful in cases like this where the last column will be variable / unknown.
.col--full {
grid-column: 1 / -1;
}
Example
Here’s the code in action. You can make any column be full width, by adding the col--full
class.
See the Pen Grid auto columns with variable sizes by Craig Fox (@craigwfox) on CodePen.
Closing thoughts
This won’t work for everything out there but it’s a handy tool to have in your grid layout toolkit. For some other layout techniques checkout my previous post on Methods for equal height columns